- C Programming Tutorial
- C Programming useful Resources
- Sample Programs In Data Structures Using Crossword
- Data Structures In C
- Simple Programs In Data Structures Using C++
C & Data Structures Page 2/174 C & Data Structures P. Kakde CHARLES RIVER MEDIA, INC. Hingham, Massachusetts. C & Data Structures. The human-readable portions of our programs to refer to data in a way that makes sense to us. To separate the computer's view of data from our own view, we use data abstraction to create other. Www.cprograms.in contains sample programs of C and Data Structures.Here you can find tutorial for many topics of C and data structures such string,array, structure,pointer,stack,queue,linked list,tree and command line argument.All these topics are explained in detail along with examples.
- Selected Reading
Arrays allow to define type of variables that can hold several data items of the same kind. Similarly structure is another user defined data type available in C that allows to combine data items of different kinds.
Structures are used to represent a record. Suppose you want to keep track of your books in a library. You might want to track the following attributes about each book −
- Title
- Author
- Subject
- Book ID
Defining a Structure
To define a structure, you must use the struct statement. The struct statement defines a new data type, with more than one member. The format of the struct statement is as follows −
The structure tag is optional and each member definition is a normal variable definition, such as int i; or float f; or any other valid variable definition. At the end of the structure's definition, before the final semicolon, you can specify one or more structure variables but it is optional. Here is the way you would declare the Book structure −
Accessing Structure Members
To access any member of a structure, we use the member access operator (.). The member access operator is coded as a period between the structure variable name and the structure member that we wish to access. You would use the keyword struct to define variables of structure type. The following example shows how to use a structure in a program −
Here I want to read and write data in the flash memory of the PIC microcontroller, ans for that I have written the following function so can you guys please check my this code and can tell me that how should I test my this code snippet. Hi-tech software picc 9.83. I have also make the EUSART functions inside it in order to check the flash functions which I have written are working correct or not.
When the above code is compiled and executed, it produces the following result −
Structures as Function Arguments
You can pass a structure as a function argument in the same way as you pass any other variable or pointer.
When the above code is compiled and executed, it produces the following result −
Pointers to Structures
You can define pointers to structures in the same way as you define pointer to any other variable − Command and conquer 3 problem with emulation software detected windows 10.
Now, you can store the address of a structure variable in the above defined pointer variable. To find the address of a structure variable, place the '&'; operator before the structure's name as follows −
To access the members of a structure using a pointer to that structure, you must use the → operator as follows −
Let us re-write the above example using structure pointer.
When the above code is compiled and executed, it produces the following result −
Sample Programs In Data Structures Using Crossword
Bit Fields
Bit Fields allow the packing of data in a structure. This is especially useful when memory or data storage is at a premium. Typical examples include −
Packing several objects into a machine word. e.g. 1 bit flags can be compacted.
Reading external file formats -- non-standard file formats could be read in, e.g., 9-bit integers.
C allows us to do this in a structure definition by putting :bit length after the variable. For example −
Here, the packed_struct contains 6 members: Four 1 bit flags f1.f3, a 4-bit type and a 9-bit my_int.
C automatically packs the above bit fields as compactly as possible, provided that the maximum length of the field is less than or equal to the integer word length of the computer. If this is not the case, then some compilers may allow memory overlap for the fields while others would store the next field in the next word.
This 'Data Structures and Algorithms In C' course is thoroughly detailed and uses lots of animations to help you visualize the concepts.
Subtitles are available for the first section only. Closed Captioning for rest of the sections is in progress and are available as [Auto-generated].
Zombie acoustic free download free. Zombie Acoustic Mp3 Download. Zombie Acoustic Mp3 Download is popular Song Mp3 in 2019, We just show max 40 MP3 list about your search Zombie Acoustic Mp3 Download Mp3, because the APIs are limited in our search system, you can download Zombie Acoustic Mp3 Download Mp3 in first result, but you must remove a Zombie Acoustic Mp3 Download from the.
This 'Data Structures and Algorithms in C' tutorial will help you develop a strong background in Data Structures and Algorithms. The course is broken down into easy to assimilate short lectures, and after each topic there is a quiz that can help you to test your newly acquired knowledge. The examples are explained with animations to simplify the learning of this complex topic. Complete working programs are shown for each concept that is explained.
This course provides a comprehensive explanation of data structures like linked lists, stacks and queues, binary search trees, heap, searching, hashing. Various sorting algorithms with implementation and analysis are included. Concept of recursion is very important for designing and understanding certain algorithms so the process of recursion is explained with the help of several examples.
This course covers following topics with C language implementation :
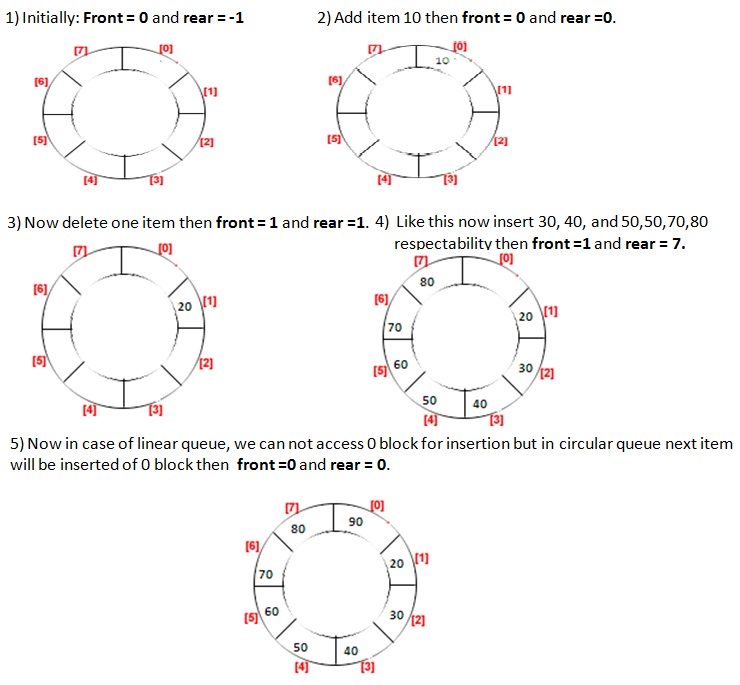
Algorithm Analysis, Big O notation, Time complexity, Singly linked list, Reversing a linked list, Doubly linked list, Circular linked list, Linked list concatenation, Sorted linked list.
Stack, Queue, Circular Queue, Dequeue, Priority queue, Polish Notations, Infix to Postfix, Evaluation of Postfix, Binary Tree, Binary Search Tree, Tree Traversal (inorder, preorder, postorder, level order), Recursion, Heap, Searching, Hashing
Sorting : Selection, Bubble, Insertion, Shell, Merging, Recursive Merge, Iterative Merge, Quick, Heap, Binary tree, Radix, Address calculation sort
Throughout the course, a step by step approach is followed to make you understand different Data Structures and Algorithms. You will see implementation of different data structures in C language and algorithms are explained in step-wise manner. Through this course you can build a strong foundation and it will help you to crack Data Structures and Algorithms coding interviews questions and work in the industry.
In this Data Structures and Algorithms course, C language is used for implementing various concepts, but you can easily implement them in any other language like C++, C#, Java, Python.
What students are saying about this course-
'This is exactly how I hoped to learn data structure and algorithm, PLUS, it's using C!!'
Is it safe to install MicroOLAP Database Designer for MySQL? Microolap database designer for mysql keygen torrent download. MicroOLAP Database Designer for MySQL was checked for possible viruses by various leading antivirus software products and it is proven to be 100% clean and safe.
'Instructor is teaching in very well and efficient manner with a good pace ,clears every doubts and teaches concepts deeply.'
'Great class, explains topics very well, better than any college class I ever took.'
'yes this course has helped me a lot in discovering new topics and the example programs are also quite helpful.'
Cpt question paper june 2009 pdf. C Tulsian, Bharat Jhunjhunwala ISBN: Fundamentals Of Accounting For CA Common Proficiency Test (CPT) Author: Tulsian ISBN: QUANTITATIVE APTITUDE: For CA Common Proficiency Test CPT Author: Kashyap Trivedi, Chirag Trivedi ISBN: Tulsian'S Key To Unlock CA-CPT Entrance Author: P. Quantitative Aptitude For CPT Author: P. Tulsian ISBN: Accountancy For CA-CPT (With CD) Author: Pathak ISBN: -> To know more about the exam online -> Any more queries, feel free to leave a post in our forum.
'I really appreciate the way the steps are broken down incrementally.'
'Deepali does a great job in explaining all the concept and the course is very well organized. First the concept is explained on paper and then there is a walkthrough of the code, and then execution of the code. I have learnt a great deal from this course.'
'I am taking notes and writing code side by side watching videos which makes it beneficial to understand the code and easier to grasp the concept of the topic rather than just copying the source code. Thank you Deepali Mam for not giving the source it was better to write the code by myself. The videos are informative, detailed and right on point with step by step code programs and I feel learned a lot taking your course then the class which I took at University. This course made my base of data structure in C pretty strong thank you for that.'
'This is an awesome course. If you need to understand then try to write every code yourself then try to analyze it. that's how you can gain confidence.'
'I'm re-learning something what I am learn years ago, and this course is perfect for my need.'
'Its quite helpfull, it nicely supplements what you have studied in the book.'
'Excellent presentation and content. Easily comprehendible .Since DS and Algorithms are heart of computer science will give a 5 star for this kind of knowledge resource.'
'It is one of the best courses that I have ever taken in DataStructures and C.'
'great way to learn !'
Data Structures In C
This Data Structures and Algorithms In C course will help software developers to refresh the concepts studied in book and also to students learning from referred book.
Simple Programs In Data Structures Using C++
- Programmers looking for jobs
- Programmers wanting to write efficient code
- Computer Science students having Data Structures as part of their curriculum
- Non Computer science students wanting to enter IT industry